Treemapping Jenkins Extension Points with R
I have been playing with R and its packages for some time, and decided to study it a bit harder. Last week I started reading the Advanced R Programming by Hadley Wickham.
One of the first chapters talks about the basic data structures in R. In order to get my feet wet I thought about a simple example: treemapping Jenkins extension points.
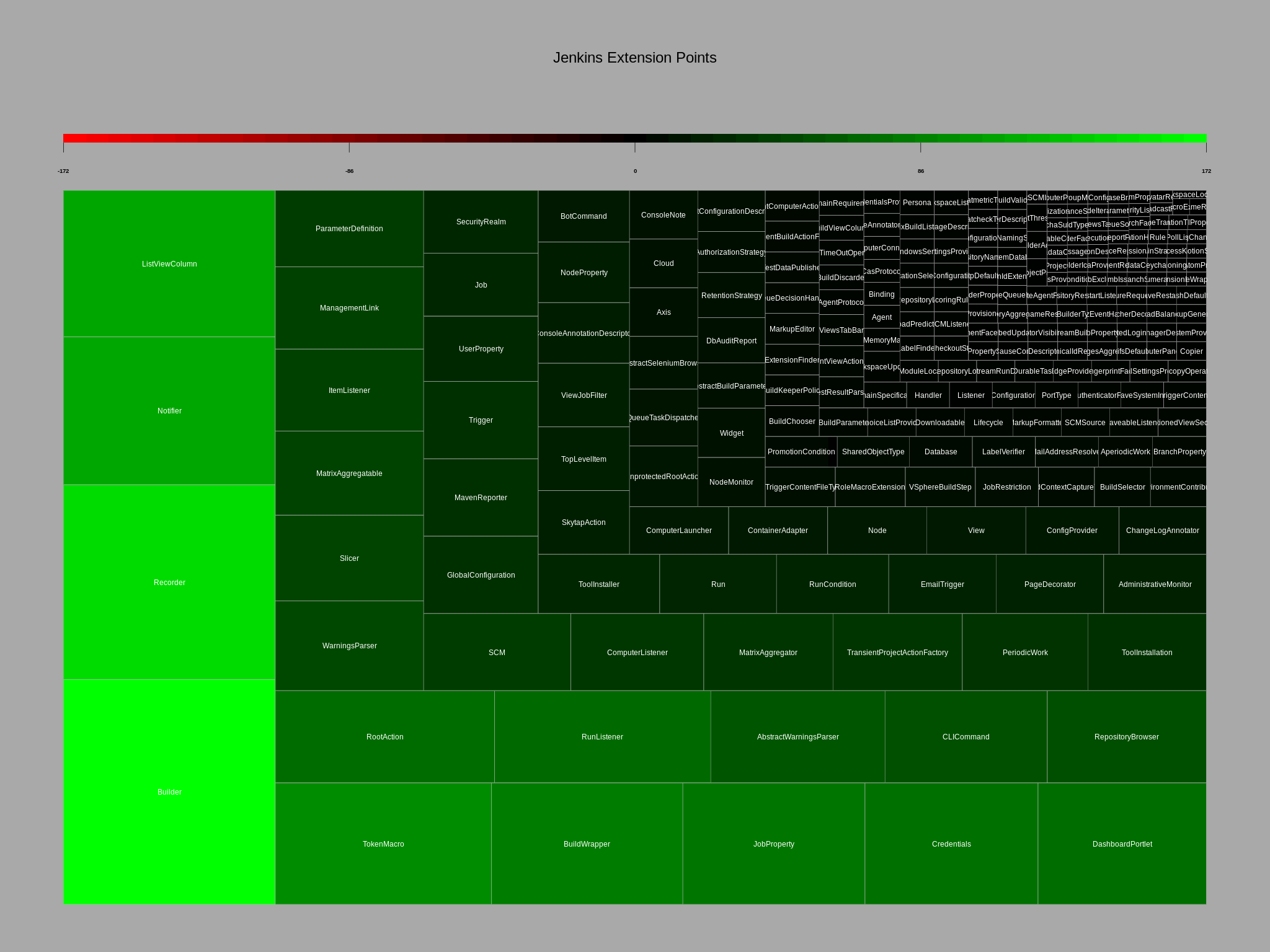
There is a Wiki page with the extension points in Jenkins (what parts of Jenkins can be customized) and its implementations. That page is generated by a Jenkins job.
That job outputs a JSON file that contains each available extension points, as well as an array with its implementations. The R code below will produce two vectors, one with the count of implementations of each extension point, and the other with the class name.
nExtensionPoints <- length(extensionPointsJson$extensionPoints)
numberOfImplementations <- vector(length = nExtensionPoints)
namesOfTheExtensionPoints <- vector(length = nExtensionPoints)
for (i in seq_along(extensionPoints)) {
extensionName = extensionPoints[[i]]$className
lastIndexOfDot = regexpr("\\.[^\\.]*$", extensionName)
namesOfTheExtensionPoints[[i]] = substr(extensionName, lastIndexOfDot[1]+1, nchar(extensionName))
numberOfImplementations[[i]] = length(extensionPoints[[i]]$implementations)
print(paste(namesOfTheExtensionPoints[[i]], " -> ", numberOfImplementations[[i]]))
}
For creating the treemap I used the portfolio package, and the map.market
function. The structures previously created and the next snippet of code are all that is
needed to create the treemap of the Jenkins extension points.
map.market(id=seq_along(extensionPoints), area=numberOfImplementations, group=namesOfTheExtensionPoints, color=numberOfImplementations, main="Jenkins Extension Points")
You can also use the Jenkins R Plug-in to produce this graph, as in this sample job. You can get the complete script in this gist, or just copy it here.
library('rjson')
library('portfolio')
download.file(url="https://ci.jenkins-ci.org/view/Infrastructure/job/infra_extension-indexer/ws/extension-points.json", destfile="extension-points.json", method="wget")
extensionPointsJson <- fromJSON(paste(readLines("extension-points.json"), collapse=""))
extensionPoints <- extensionPointsJson$extensionPoints
nExtensionPoints <- length(extensionPointsJson$extensionPoints)
numberOfImplementations <- vector(length = nExtensionPoints)
namesOfTheExtensionPoints <- vector(length = nExtensionPoints)
for (i in seq_along(extensionPoints)) {
extensionName = extensionPoints[[i]]$className
lastIndexOfDot = regexpr("\\.[^\\.]*$", extensionName)
namesOfTheExtensionPoints[[i]] = substr(extensionName, lastIndexOfDot[1]+1, nchar(extensionName))
numberOfImplementations[[i]] = length(extensionPoints[[i]]$implementations)
print(paste(namesOfTheExtensionPoints[[i]], " -> ", numberOfImplementations[[i]]))
}
png(filename="extension-points.png", width=2048, height=1536, units="px", bg="white")
map.market(id=seq_along(extensionPoints), area=numberOfImplementations, group=namesOfTheExtensionPoints, color=numberOfImplementations, main="Jenkins Extension Points")
dev.off()
Categories: Blog